Go语言 :使用简单的 for 迭代语句进行 TDD 驱动测试开发与 benchmark 基准测试
for
用来循环和迭代,while
,do
,until
这几个关键字,我们只能使用 for
。这也算是件好事!先编写测试用例
package iteration import "testing" func TestRepeat(t *testing.T) { repeated := Repeat("a") expected := "aaaaa" if repeated != expected { t.Errorf("expected '%q' but got '%q'", expected, repeated) } }
先使用最少的代码来让测试先跑起来
package iteration func Repeat(character string) string { return "" }
把代码补充完整,使得它能够通过测试
func Repeat(character string) string { var repeated string for i := 0; i < 5; i++ { repeated = repeated + character } return repeated }
就像大多数类 C 的语言一样,for
语法很不起眼。
与其它语言如 C,Java 或 JavaScript 不同,在 Go 中 for
语句前导条件部分并没有圆括号,而且大括号 { } 是必须的。你可能会好奇下面这行
var repeated string
我们目前都是使用 :=
来声明和初始化变量。然后 :=
只是简写(简短模式定义看这里??)。这里我们使用显式的版本来声明一个 string
类型的变量。我们还可以使用 var
来声明函数,稍后我们将看到这一点
运行测试应该是通过的
重构
现在是时候重构并引入另一个构造(construct)+=
赋值运算符。
const repeatCount = 5 func Repeat(character string) string { var repeated string for i := 0; i < repeatCount; i++ { repeated += character } return repeated }
+=
是自增赋值运算符(Add AND assignment operator),它把运算符右边的值加到左边并重新赋值给左边。
它在其它类型也可以使用,比如整数类型。
基准测试
在 Go 中编写基准测试(benchmarks)是该语言的另一个一级特性,它与编写测试非常相似。
func BenchmarkRepeat(b *testing.B) { for i := 0; i < b.N; i++ { Repeat("a") } }
你会看到上面的代码和写测试差不多。
testing.B
可使你访问隐性命名(cryptically named)b.N
。
b.N
次,并测量需要多长时间。go test -bench=.
来运行基准测试。 (如果在 Windows Powershell 环境下使用 go test -bench="."
)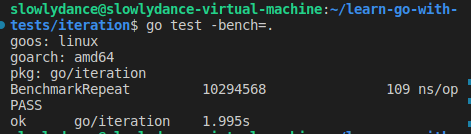
以上结果说明运行一次这个函数需要 109 纳秒(在我的电脑上)。这挺不错的,为了测试它运行了 10294568 次
注意:基准测试默认是顺序运行的。
以上
本文来自博客园,作者:slowlydance2me,转载请注明原文链接:https://www.cnblogs.com/slowlydance2me/p/17232808.html